Developing web applications in Java is a way to create high-performance digital platforms. This guide explains the steps of creating Java web applications, demonstrating the reliability and adaptability of Java web application code. Whether you want to develop a Java web application yourself or are considering hiring a Java developer, understanding Java’s basic elements and benefits in web development is paramount.
- Java offers platform independence for web development, reducing code rewriting.
- Java’s modular architecture provides flexibility and scalability for complex web apps.
- Java’s rich APIs, security features, and community support enhance development.
What is a Java Web Application?
A Java web application is a dynamic platform allowing developers to build a Java web application using Java web application code. These applications serve interactive user experiences through browsers. Crafting a web app in Java involves utilizing Java web application code, ensuring robust functionality and responsiveness. Java web application development emphasizes creating efficient, scalable web app Java solutions.
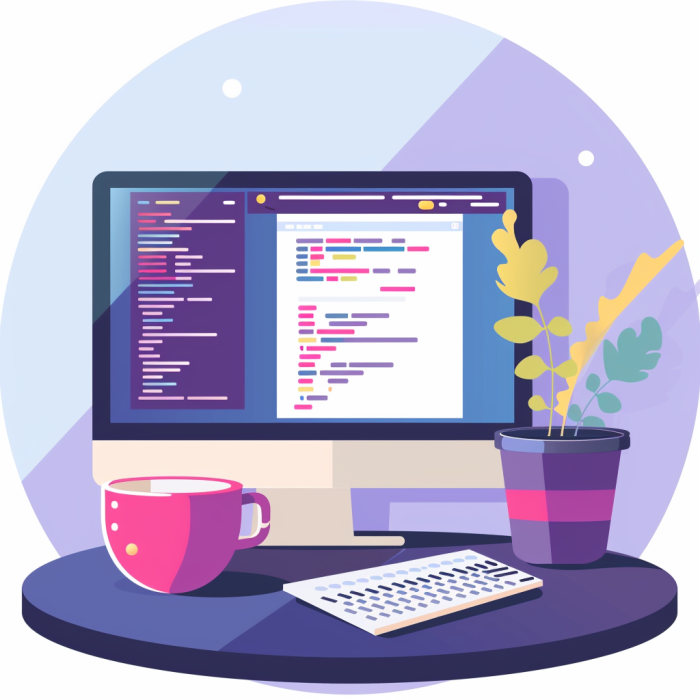
Why Choose Java for Web Applications Development
Choosing Java for web application development is a profitable step for many developers. The ability to build a Java web application that’s platform-independent and secure, coupled with Java’s web application code, rich APIs, and extensive community support, makes web app Java an attractive choice. Moreover, memory management and scalability efficiency ensure that a Java web application stands out in the digital domain.
- Platform Independent
Java’s platform independence is a major Java web application development advantage. Being platform-independent means developers can write Java web application code once and deploy it across various platforms without modification. This flexibility allows for easier scaling and updating of a Java web app, making building a Java web application more efficient and cost-effective.
- Flexible & Scalable
Java web application development stands out for its inherent flexibility and scalability. Developers who build a Java web application benefit from the language’s ability to adapt and grow with the application’s demands. Whether scaling up for more users or expanding functionality, Java’s web app’s Java architecture supports it. This makes Java web application code ideal for evolving projects, ensuring long-term effectiveness.
- Rich Set of APIs
Using Java to develop web applications provides access to a wide range of APIs that make it easy to create Java web applications. Developers who want to create a Java web application can use these APIs for various tasks, including I/O operations, XML parsing, and database connections, making Java web application code more robust and versatile. This abundance of resources supports a wide range of functionality for any Java web application project.
- Highly Secure
When you develop web applications in Java, you put security first. Java allows developers to integrate advanced features such as secure login, custom security policies, and cryptography into their Java web application projects. The ability to build Java web applications using digital signatures and ciphers emphasizes that Java web application code is inherently secure, making Java the best choice for building secure web applications.
- Responsive IDEs & Tools
The availability of responsive IDEs and tools greatly enhances building a Java web application. Java web application development benefits from robust IDEs like NetBeans and Eclipse, streamlining web app Java creation and testing. Along with numerous open-source plugins, these tools simplify Java web application code development, making it easier for developers to craft and refine their Java web applications efficiently.
- Multi-Thread Support
Java web application development excels with multi-thread support, enabling web app Java to manage multiple users and threads simultaneously. This feature allows developers to build a Java web application without duplicating Java web application code for concurrent operations. The multi-threaded capability of Java ensures a Java web application offers faster response times, enhanced performance, and efficient handling of multiple operations, making it a robust choice for developers.
- Wide Community Support
The Java web application development community is vast, providing unparalleled support for those who build a Java web application. This wide community support means web app Java developers have access to a plethora of resources, including forums, groups, and organizations dedicated to Java web application code. Assistance is readily available, making it easier for new and seasoned developers to navigate challenges and enhance their Java web application projects.
Java Technologies Used in Web Application Development
Developing web applications in Java involves using various technologies, although the wide range may seem overwhelming at first. It’s essential to realize that not every web app Java endeavor necessitates using all available tools. A Java web application can be as straightforward as a single page crafted with JavaServer Pages (JSP), or it may integrate several technologies. The key is understanding the tools at your disposal and selecting the appropriate ones to build a Java web application effectively, using the most suitable Java web application code.
Java IDE
Java IDEs are essential for developers building a Java web application efficiently. These environments provide comprehensive tools for writing, testing, and debugging Java web application code. Popular choices like Eclipse and IntelliJ IDEA enhance productivity, allowing for rapid development of web app Java projects. They integrate various technologies, simplifying the process of building a Java web application and making them indispensable for Java web application developers.
Java Servlet API
The Java Servlet API provides a robust foundation for web app Java development, enabling the creation of dynamic, server-side Java web applications. This API includes interfaces like Servlet and servletconfig, facilitating tasks from data display to form processing. Servlets, pivotal in processing requests and interacting with databases, are instrumental in building Java web applications that are both efficient and platform-independent. Developers can craft responsive Java web application code through servlets, making it a cornerstone of Java-based web solutions.
Java Servlet API Example
Objective: Process a simple GET request and respond with a personalized greeting message.
Servlet Class Name: GreetingServlet.java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
import java.io.PrintWriter;
public class GreetingServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Set response content type
response.setContentType("text/html");
// Get the writer to respond
PrintWriter out = response.getWriter();
// Get user name from request parameters
String name = request.getParameter("name");
if(name == null) name = "Guest";
// Generate the HTML content
out.println("<html><head><title>Greeting</title></head><body>");
out.println("<h2>Hello, " + name + "!</h2>");
out.println("</body></html>");
}
}
JavaServer Pages (JSP)
JavaServer Pages (JSP) technology revolutionizes the development of dynamic content for Java web applications, offering a blend of static and dynamic document elements. This technology simplifies embedding Java code into HTML, making it easier to build Java web applications. With JSP, developers gain access to Java’s full capabilities, enabling the creation of intricate and responsive web app Java interfaces. JSP’s integration of servlet code into web pages enhances the Java web application code, streamlining the development process and boosting productivity.
JavaServer Pages (JSP) Example
Objective: Display the current date and time on a web page using JSP.
Filename: currentDateTime.jsp
<%@ page import="java.util.Date" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Current Date and Time</title>
</head>
<body>
<h2>Current Date and Time:</h2>
<%
Date now = new Date();
out.println(now.toString());
%>
</body>
</html>
JSP Standard Tag Library
JSP Standard Tag Library (JSTL) simplifies Java web application development by offering a collection of custom tags, streamlining the Java web application code. Developers can avoid Java code in JSP files, promoting cleaner designs and easier maintenance of web app Java projects. JSTL enhances code readability and reduces development time, making it easier to build a Java web application.
For the JSP Standard Tag Library (JSTL), here’s an example of using JSTL to iterate through a list of items in a Java web application:
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<body>
<h2>List of Items</h2>
<ul>
<c:forEach var="item" items="${items}">
<li>${item}</li>
</c:forEach>
</ul>
</body>
</html>
This JSTL code snippet demonstrates how to dynamically generate HTML list items based on the items attribute available in the request scope, simplifying the Java web application code.
JavaServer Faces
JavaServer Faces (JSF) revolutionizes Java web application creation by providing a robust framework for building component-based UIs. It simplifies Java web application code management, offering a rich library of UI components for web app Java development. JSF’s event-driven programming model and integrated development environment support make it an excellent choice for developers aiming to build a Java web application with complex interfaces.
For JavaServer Faces (JSF), here’s an example of a simple JSF page that uses JSF UI components to build a form in a Java web application:
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html">
<h:head>
<title>Simple JSF Form</title>
</h:head>
<h:body>
<h3>Feedback Form</h3>
<h:form>
<h:outputLabel for="name" value="Name:" />
<h:inputText id="name" value="#{userBean.name}" />
<h:commandButton value="Submit" action="response" />
</h:form>
</h:body>
</html>
This JSF code snippet creates a simple form with a label, an input field for the name, and a submit button. The input field is bound to a managed bean property name in the Java web application code, demonstrating how JSF facilitates linking UI components to Java web application data models.
JAXP
JAXP enhances Java web application development by offering tools to parse and transform XML documents, which is crucial for web app Java functionality. It supports DOM, SAX, and XSLT, allowing developers to build a Java web application with sophisticated XML handling capabilities. JAXP’s flexibility in parser and XSL processor choice simplifies incorporating complex XML java web application code, making it indispensable for robust Java web application development.
JAXP Example
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import java.io.File;
public class JAXPExample {
public static void main(String[] args) {
try {
File inputFile = new File("input.xml");
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(inputFile);
doc.getDocumentElement().normalize();
System.out.println("Root element :" + doc.getDocumentElement().getNodeName());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Java Persistence API
Java Persistence API (JPA) revolutionizes data management in Java web application development. By mapping object-oriented models to databases, JPA simplifies persisting data in web app Java projects. It eradicates the need for complex SQL in Java web application code, making it easier to build a Java web application. JPA’s efficient class and method set ensure smooth database integration, enhancing Java web application performance.
Java Persistence API Example
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Persistence;
public class JPAExample {
public static void main(String[] args) {
EntityManagerFactory emf = Persistence.createEntityManagerFactory("persistenceUnit");
EntityManager em = emf.createEntityManager();
em.getTransaction().begin();
User user = new User();
user.setName("John Doe");
user.setEmail("[email protected]");
em.persist(user);
em.getTransaction().commit();
em.close();
emf.close();
}
}
Note: This JPA example assumes you have a User entity defined and a persistence unit named “persistenceUnit” configured in your persistence.xml.
JDBC API
JDBC API is fundamental for Java web application development, enabling direct database interaction. It provides a variety of drivers for web app Java connectivity, facilitating operations like data retrieval and updates. Incorporating JDBC API in Java web application code allows developers to build a Java web application with dynamic data access. Its support for SQL enhances Java web application functionality, ensuring efficient database communication.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class JDBCExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/databaseName";
String user = "username";
String password = "password";
try {
Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM tableName");
while (rs.next()) {
System.out.println(rs.getString("columnName"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
JDBC API Example
Note: For the JDBC example, ensure you have the appropriate JDBC driver in your classpath, and replace databaseName, username, password, tableName, and columnName with your actual database details.
Ready to embark on your Java web app development journey?
Contact UsSteps to Develop a Web Application Using Java
Developing a Java web application involves a structured approach that begins with setting up the essential tools: Java, an IDE like Eclipse or Netbeans, a server such as Tomcat for Servlets, and a database like MySQL or Oracle. Building a Java web application unfolds through seven key steps, ensuring your web app Java project transitions from concept to reality efficiently. This section describes how to write Java web application code efficiently.
Step 1: Open Eclipse Create a Dynamic Web Project
Open Eclipse and initiate a Dynamic Web Project. Navigate through File > New, opt for Dynamic Web Project, and ensure the generation of web.xml for your project setup. This action establishes the initial structure required to build a Java web application effectively.
Step 2: Provide Project Name
Assigning a project name is a straightforward process critical for organizing your Java web application development. Input a project name that reflects its functionality or purpose, and generate a web.xml deployment descriptor, which is essential for defining the structure of your web app, Java.
Step 3: Create a Servlet
To add functionality to your Java web application, create a Servlet by right-clicking on the Java Resources/src folder and selecting New > Servlet. Provide a name for your Servlet, which acts as a request handler within your application, enriching your web app Java with dynamic content capabilities.
Step 4: Add the Servlet Jar file
Integrating the Servlet JAR file into your project is necessary for the servlet to function correctly. Access the project’s Build Path settings and add the servlet-api.jar file via the Add External JARs option. This inclusion equips your project with the necessary libraries to execute servlets within your Java web application.
Step 5: Create an HTML or JSP file
Developing an HTML or JSP file is essential for creating the user interface of your Java web application. This file, placed within the WebContent directory, provides the visual and interactive elements that users will engage with, making it a fundamental component of your web app, Java.
Step 6: Map the File
Linking your servlet to the HTML or JSP file through servlet mapping ensures that user requests are directed appropriately. This configuration, done within the web.xml file or through annotations in your servlet, establishes the connection between your application’s front-end and back-end components, enhancing the functionality of your Java web application.
Step 7: Run the Application
The final step involves launching your Java web application to verify its operation. Employing Eclipse’s server or an external server like Tomcat allows you to run your application and interact with and test the features and functionalities you’ve implemented in your Java web application code.
Examples of Java Web Applications
Exploring Java web application examples offers invaluable insights into how to build a Java web application effectively. These instances illuminate diverse strategies for crafting web app Java solutions, showcasing varied functionalities and Java web application code structures that cater to different business needs and user experiences.
Spotify
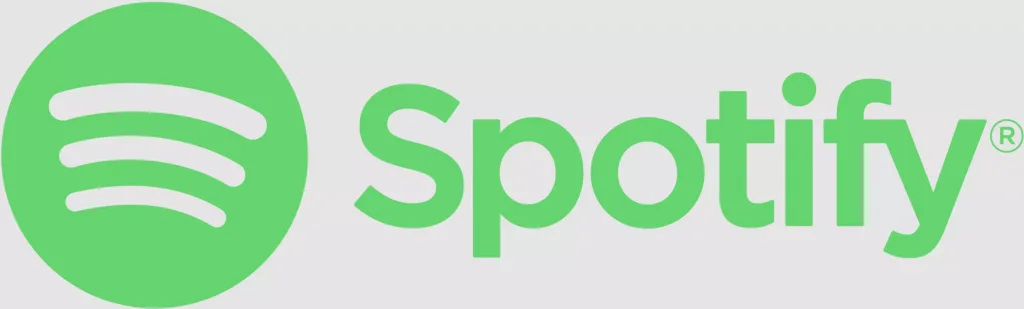
Spotify, a premier Java web application for music streaming, showcases the power of Java web application code in delivering a vast library of tunes and podcasts to a global audience. This web app, the Java platform, enhances user experience, ensuring accessibility across devices, thanks to Java’s versatility. Spotify revolutionizes music discovery and sharing, solidifying its position in the digital music ecosystem.
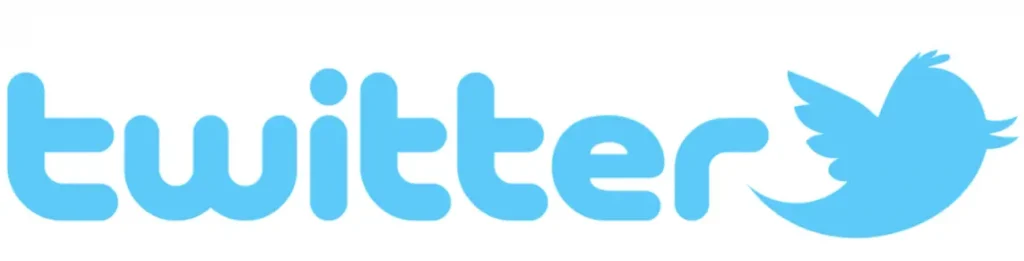
Twitter is a pivotal Java web application in social networking, using Java web application code. This web app, Java, enables real-time sharing of concise content, engaging a vast user base. Twitter’s Java foundation facilitates rapid information exchange and connectivity, making it a dynamic global discourse and entertainment platform.
Opera Mini
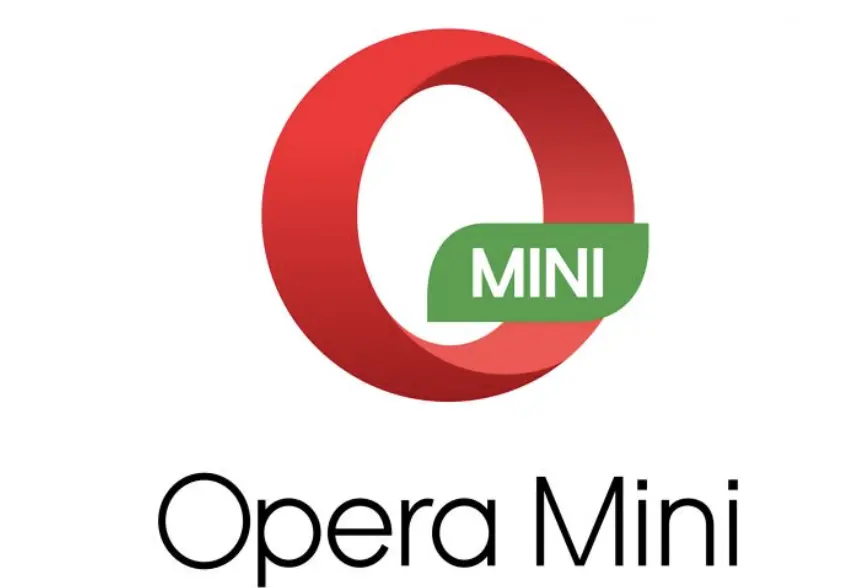
Opera Mini, a Java web application, redefines mobile web browsing efficiently and quickly. Leveraging Java web application code, this web app Java optimizes data usage without compromising performance, ideal for users with limited internet access. Opera Mini’s Java-based technology ensures a seamless browsing experience, emphasizing Java’s role in innovative web solutions.
Nimbuzz Messenger
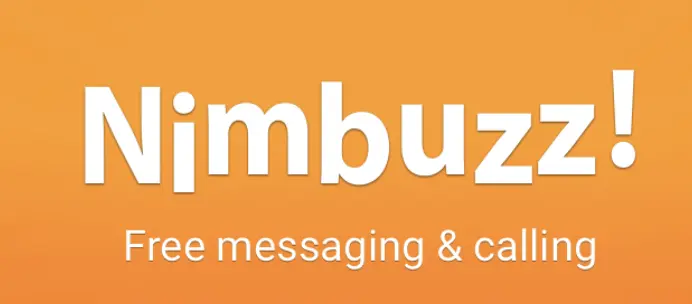
Nimbuzz Messenger exemplifies the versatility of Java web application code in communication apps. As a Java web application, it bridges users globally through instant messaging and calls, highlighting Java’s capability to support cross-platform web app Java services. Nimbuzz’s Java foundation caters to a diverse user base, emphasizing Java’s significance in global connectivity.
CashApp
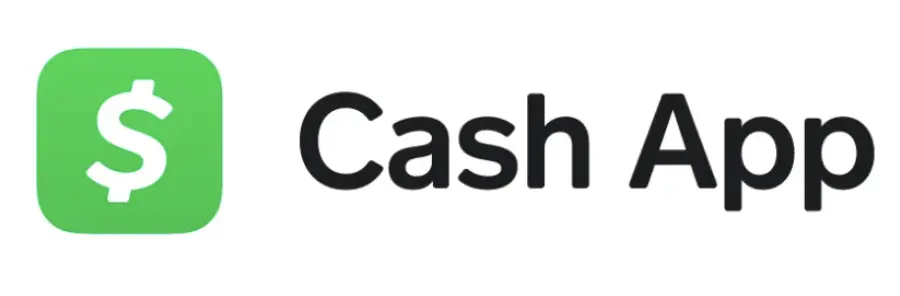
CashApp leverages Java web application code to redefine financial transactions, making it a standout Java web application for mobile payments. This web app, Java, ensures secure, instant money transfers, showcasing Java’s reliability in handling sensitive data. CashApp’s Java-powered framework highlights the importance of Java in the fintech sector, offering convenience and security to users worldwide.
Final Thoughts
Delving into the creation of a Java web application offers an enriching path for developers aiming to craft cutting-edge digital solutions. Mastering the Java web app and Java web application code is essential for those looking to build a Java web application that stands out in the competitive digital space. This knowledge not only equips developers with the tools needed for sophisticated web development but also enhances their applications’ functionality and user experience.
Ficus Technologies specializes in transforming ideas into reality through expert Java web application development. Our team excels in crafting bespoke solutions tailored to our client’s unique needs.
Java offers several key advantages for web app development, including platform independence, a flexible and scalable modular architecture, a rich set of APIs, robust security features, responsive IDEs, and multi-thread support. Its wide developer community provides valuable support, making Java an ideal choice for creating versatile and efficient web applications.
Java’s platform independence allows developers to write code once and run it on any platform with a Java Virtual Machine (JVM). This eliminates the need for rewriting code for different operating systems, streamlining the development process. It provides a consistent user experience across various platforms, making Java a versatile and practical choice for web development.