Mastering regular expressions in Python equips you with a vibrant tool set for examining and controlling text information. These expressions, a sequence of characters creating search patterns, allow you to efficiently match, extract, and modify text strings across different datasets. Python’s re-module improves this capability by providing robust functions that sustain intricate text operations. This makes Python a perfect platform for discovering and using routine expressions, allowing you to handle even the most complicated text-handling tasks efficiently.
- Regular expressions streamline complex text manipulation and data retrieval.
- Mastery of regex boosts efficiency in various programming tasks.
- Python’s regex tools are essential for advanced data analysis.
What is a Regular Expression?
A Python regular expression is a powerful tool for pattern discovery and text adjustment. It utilizes a collection of characters that specify a search pattern essential for information extraction and recognition tasks. For example, it can recognize all e-mail addresses in a text or confirm the correct format for the contact number. With Python’s re-module, these patterns permit innovative text analysis and modifications, supporting a variety of procedures from easy searches to complicated text transformations.
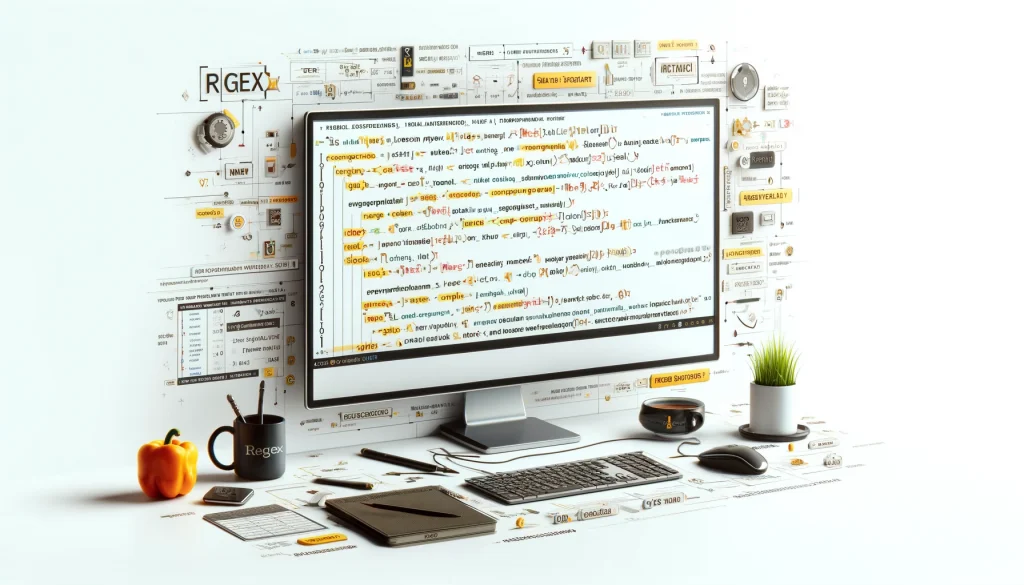
Python Module for Regular Expression
The Python regular expression module, known as re, is crucial for carrying out regular expressions in Python. This module gears up users with tools to carry out searches, substitutes, and splits in strings using regex patterns. It streamlines complex string procedures, making tasks like data parsing and validation uncomplicated. Its functionality consists of putting together regex patterns for performance, looking for patterns, and drawing out or replacing texts, indispensable for effective text processing and data manipulation tasks.
We chose to use python because we wanted a well-supported scripting language that could extend our core code. Indeed, we wrote much more code in python than we were expecting, including all in-game screens and the main interface.
Soren Johnson
Using Regular Expressions in Python
Why should you learn to utilize regular expression Python capacities properly? Python’s built-in re-module provides durable assistance for parsing and adjusting strings through routine expressions, assisting in tasks like searching, data recognition, and complicated text controls. How can mastering these tools improve your coding jobs? The subsequent paragraphs delve into the re-module’s functions and special characters, providing sensible insights into their functional applications.
. – Dot
In Python regular expression syntax, the dot (.) matches any single character except the newline character (\n). For example, the pattern ‘a.b’ can match ‘acb’, ‘arb’, or ‘a3b’, among others, since the dot represents any character between ‘a’ and ‘b’. Similarly, ‘..’ checks if a string contains at least two characters, with each dot representing a different character. This allows for flexible searching and manipulation of text strings.
* – Star
The star (*) in Python, regular expression syntax, allows for matching zero or more occurrences of the preceding element. For example, the pattern ‘abc’ matches ‘ac’, ‘abc’, ‘abbc’, ‘abbbc’, and so on, capturing instances where ‘b’ may not appear or appear multiple times before a ‘c’. However, it will not match ‘abdc’, where ‘d’ disrupts the sequence. This versatility enables precise control over text parsing, greatly enhancing data manipulation and analysis capabilities.
+ – Plus
The plus (+) sign in Python regular expression syntax matches one or more occurrences of the element preceding it. For instance, ‘ab+c’ can match ‘abc’, ‘abbc’, or ‘abbbc’, as it requires at least one ‘b’ followed by ‘c’. It will not match ‘ac’, where ‘b’ is absent, nor ‘abdc’, where ‘d’ interrupts the required sequence. This feature allows for flexible yet controlled searching within strings, efficiently extracting and analyzing varying patterns.
? – Question
The question mark (?) in Python regular expression syntax makes the preceding element optional, matching either once or not. For example, ‘ab?c’ will match ‘ac’, which skips ‘b’, and ‘abc’, which includes one ‘b’. It also matches within longer strings like ‘dabc’. However, it does not match ‘abbc’ with two ‘b’s or ‘abdc’, where ‘d’ disrupts the pattern. This operator is useful for flexible string searching, accommodating variations in data formats or textual input.
Braces {m, n}
Braces in regular expression types in Python specify a range of repetitions for the preceding element. For example, the pattern ‘a{2,4}’ matches strings containing ‘a’ repeated two to four times. It effectively identifies matches like ‘aaab’, ‘baaac’, and ‘gaad’, where ‘a’ appears within the defined range. However, it will not match ‘abc’ or ‘bc’, where ‘a’ appears fewer than two times or not, making this tool adept at handling precise text-matching requirements in data parsing tasks.
Square brackets [].
In regular expressions in Python, square brackets ([]), create a character class to match specific characters. For instance, [abc] matches any single ‘a’, ‘b’, or ‘c’. With the ‘-‘ symbol, ranges of characters can be specified, like [0-3] for numbers 0 to 3, or [a-c] for letters ‘a’ to ‘c’. Adding a caret (^) negates the character class. For example, [^0-3] matches any number except 0, 1, or 3, while [^a-c] matches any character except ‘a’, ‘b’, or ‘c’. This versatility enables precise pattern matching in text-processing tasks
\ Backslash
In regular expression Python coding, the backslash (/) is crucial for specifying that subsequent characters should be treated literally, not as metacharacters. For example, a period (.) normally matches any character. However, prefixing it with a backslash (/), changes its behavior to match only the period character. Consider the code below:
import re
s = 'suman.singh'
# without using backslash
match = re.search(r'.', s)
print(match) # Outputs: <re.Match object; span=(0, 1), match='s'>
# using backslash
match = re.search(r'\.', s)
print(match) # Outputs: <re.Match object; span=(6, 7), match='.'>
This unlikeness is important for acquiring accurate search results in data parsing tasks.
| – Or Symbol
The logical OR operator is the vertical bar or pipe symbol (|) in regular expressions in Python. This functionality allows for matching any of multiple possible patterns within a text. For example, the pattern a|b will successfully match strings that include either ‘a’ or ‘b’, such as ‘acd’, ‘bcd’, or ‘abcd’. This operator is particularly useful for scenarios where multiple potential matches must be recognized within strings, enhancing flexibility and efficiency in pattern recognition and data extraction.
Dive into Python Regular Expressions with Ficus Technologies!
Contact UsBasic Regular Expression Operations
Comprehending these fundamental tools helps you control and search text properly. The following sections dive deeper into patterns, matches, and much more, allowing Python developers to take advantage of regular expressions in Python for practical applications, from data validation to parsing complex text inputs. Let’s explore how these operations develop the bedrock of text handling.
1. Searching for Matches
Using regular expression Python, the re.search function from the re module is employed to identify if a given pattern appears within a string. For example, if you want to determine whether the word “welcome” exists in the sentence “Hello, welcome to the world of Python!”, the code snippet would look like this:
import re
text = "Hello, welcome to the world of Python!"
pattern = "welcome"
result = re.search(pattern, text)
if result:
print("Pattern found!")
else:
print("Pattern not found.")
This method effectively filters and identifies specific sequences in larger text bodies, enhancing data parsing and validation processes.
2. Replacing Matches
Using regular expression Python, the re.sub function efficiently replaces specific substrings within a text. For instance, to update terms in an instructional document, you could replace the word “teacher” with “instructor” across the entire text. Here’s a practical code example:
import re
text = "The teacher will review the chapter."
pattern = "teacher"
replacement = "instructor"
new_text = re.sub(pattern, replacement, text)
print(new_text)
This script outputs “The instructor will review the chapter.”, demonstrating the power of pattern replacement for text editing and content management tasks.
3. Splitting a String Based on a Pattern
Using regular expression Python, the re.split function facilitates the division of strings into lists based on defined patterns. For example, separating elements in a data string formatted with commas becomes straightforward. Here’s how to execute this:
import re
data = "apple,banana,cherry"
pattern = ","
elements = re.split(pattern, data)
print(elements)
This code splits the string “apple,banana,cherry” into [“apple”, “banana”, “cherry”], making it useful for parsing comma-separated values in data processing tasks.
4. Regular Expression Flags
Regular expression Python functionality includes using flags that adjust how patterns are matched. For instance, the re.DOTALL flag also allows the dot (.) metacharacter to match newline characters, which is useful in data extraction scenarios spanning multiple lines. Here’s an application:
import re
text = "First line.\nSecond line."
pattern = "First line.*Second line"
# Search without re.DOTALL would fail as it doesn't match across new lines
result = re.search(pattern, text, re.DOTALL)
if result:
print("Match found across lines")
else:
print("No match found")
This script confirms a match across different lines, demonstrating how flags modify matching behaviors in diverse contexts.
5. Grouping and Capturing
Regular expression Python allows for effective substring extraction through grouping and capturing. Consider this example:
import re
text = "John Doe: 30, Jane Smith: 25"
pattern = "(\w+ \w+): (\d+)"
# Find all matches and capture groups
results = re.findall(pattern, text)
for name, age in results:
print(f"{name} is {age} years old")
This code extracts names and ages from a string, demonstrating grouping in regular expressions. The final method captures each name-age pair, enabling streamlined data parsing.
Use Cases of Regular Expressions
What makes regular expression Python tools so indispensable across various programming tasks? Regular expressions offer powerful solutions for data validation, parsing, and transformation challenges. Next, we’ll look at specific examples of regular expressions to show how they can be used to improve search capabilities, automate data mining operations, and speed up complex text manipulation, all of which have real-world applications.
Identify the Patterns to Get the Name and Age
Utilizing regular expression Python tools efficiently enables extracting organized info like names and ages from unstructured text data. The complying with Python bit shows just how to make use of routine expressions to recognize patterns and analyze a string consisting of names beginning with capital letters) and ages (stood for by numbers). This approach improves data processing tasks, specifically in situations entailing text analysis or data removal from documents.
import re
# Example text
text_data = "Alice is 30, Bob is 45, and Charlie is 25"
# Regular expressions to find names and ages
names = re.findall(r'[A-Z][a-z]+', text_data)
ages = re.findall(r'\d+', text_data)
# Combining names and ages into a dictionary
name_age_dict = dict(zip(names, ages))
print(name_age_dict)
Output:
{'Alice': '30', 'Bob': '45', 'Charlie': '25'}
This code neatly associates names with their respective ages, demonstrating the practical utility of regular expression types in Python for structured data extraction.
Email Validation
Email validation is a usual necessity in software program advancement, where regular expression Python tools can be used to guarantee that individual input conforms to expected email format standards. The following with Python code bit highlights how to validate e-mails using a Python regular expression. It focuses on validating that the e-mail consists of appropriate segments for a username, domain name, and top-level domain name, therefore boosting data integrity and customer communication quality.
import re
# List of example emails
emails = ['[email protected]', '[email protected]', '[email protected]']
# Regular expression for validating an email
email_pattern = r'^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$'
# Checking each email against the pattern
for email in emails:
if re.match(email_pattern, email):
print(email, ': valid')
else:
print(email, ': invalid')
Output:
[email protected] : valid
[email protected] : invalid
[email protected] : valid
This code effectively demonstrates the utility of regular expressions in validating email formats, ensuring they meet the criteria before being processed or stored.
Obtaining Details From the Address Text
Removing particular information from address strings can enhance information processing and improve database administration. One can successfully analyze and classify each part of an address using regular expression Python. Below is a Python code snippet demonstrating exactly how to utilize a Python regular expression to extract apartment numbers, street names, and city, state, and postal codes from address strings:
import re
# Example address string
addresses = [
"Apartment 19, 123 Baker Street, Gotham, NY, 10001",
"Unit 5, 987 Elm Street, Metropolis, IL, 62901"
]
# Regular expression pattern for extracting address components
address_pattern = r"(Apartment \d+|Unit \d+), (\d+ \w+ Street), (\w+), ([A-Z]{2}), (\d{5})"
# Extract and print address components
for address in addresses:
match = re.search(address_pattern, address)
if match:
print("Apartment/Unit:", match.group(1))
print("Street:", match.group(2))
print("City:", match.group(3))
print("State:", match.group(4))
print("Zip Code:", match.group(5))
print("---")
This code identifies and separates different address components, facilitating easier data manipulation and validation.
Final Words
Mastering regular expressions in Python equips customers to search, edit, and adjust text efficiently. This capability is particularly valuable in environments requiring accurate data extraction and analysis. Developers can simplify intricate string operations through Python’s regular expression library, minimize development time, and boost software efficiency. Regular expressions enable accurate data parsing, which is important in fields like information validation, natural language processing, and server log analysis.
Ficus Technologies can integrate these capacities to improve their text handling systems. Installing Python regular expressions allows Ficus Technologies to automate data extraction procedures, making them faster and more dependable. This directly adds to functional performance and accuracy in data management.
Python supports regular expressions through its built-in library called re, which provides functions for various string pattern-matching tasks. Using this module, users can compile regular expressions into objects for efficiency, search for patterns within strings, replace substrings, and split strings based on regex patterns. Functions like re.search(), re.match(), re.findall(), and re.sub() facilitate these operations. Python’s regex capabilities extend to support advanced pattern-matching features like lookahead and lookbehind assertions and modifiers to change how patterns are interpreted, such as case insensitivity and multiline matching. This makes Python a robust tool for text processing.
In Python, regular expressions can be accessed using the re-module, part of the standard library. To utilize it, you must first import the module with import re. Once imported, you can access the module’s various functions to perform different operations involving regular expressions. For instance, to find all matches of a pattern, you use re.findall(pattern, string), to search for a match at the beginning of a string, you use re.match(pattern, string), and to search anywhere within the string, you use re.search(pattern, string). Patterns are typically defined as raw strings using r”text”.